Introduction to Python Exceptions
Exception handling is a critical part of programming that allows developers to elegantly resolve unforeseen mistakes while maintaining program stability. Exceptions in Python are occurrences that occur during program execution that disturb the usual flow of instructions. Understanding how to handle exceptions is critical for developing strong and dependable programs.
Brief Explanation of Exceptions in Python
Exceptions are raised in Python when an error occurs during program execution. This might be due to a number of factors, including improper input, a missing file, or division by zero. When an exception occurs, Python creates an exception object containing details about the issue, and the program’s usual flow is disrupted.
Why Handling Exceptions is Important in Programming
Handling exceptions is critical for a number of reasons. For starters, it prevents your software from crashing unexpectedly, allowing for controlled recovery. Second, it aids in the identification and resolution of possible concerns throughout development. Finally, it improves the overall user experience by displaying useful error messages instead of cryptic tracebacks.
Basic Exception Handling
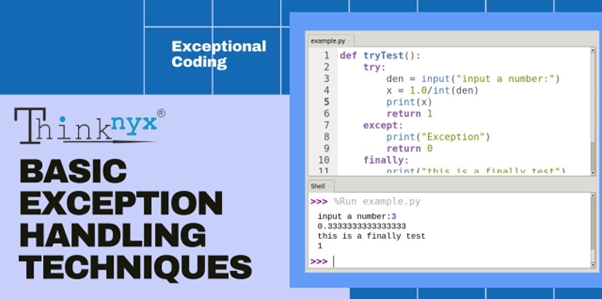
Syntax of a try-except Block
The basic building element for managing exceptions in Python is the try-except block. The code that might throw an exception is included in the try block, and the code to handle the exception is contained in the except block.

python
try:
# Code that may raise an exception
except ExceptionType as e:
# Code to handle the exception
- ‘try’ : This block contains the code that may raise an exception. It’s the portion of your code where you anticipate the possibility of an error occurring.
- ‘except ExceptionType as e:’: This block specifies the type of exception you want to catch and handle. If an exception of type ExceptionType or any of its subclasses is raised in the try block, the code within this except block will be executed. The as e part allows you to reference the exception object using the variable e within the except block.
Handling Specific Exceptions Using Except Clauses
To handle various kinds of exceptions, you can use numerous except clauses. This enables you to customize your answer according to the particular mistake that took place.

python
try:
#Code that may raise an exception
except ValueError as ve:
# Handle ValueError
print(f”Caught a ValueError: {ve}”)
except FileNotFoundError as fe:
# Handle FileNotFoundError
print(f”Caught a FileNotFoundError: {fe}”)
Using the Else Block in Exception Handling
If there are no exceptions thrown in the try block, the else block is invoked. It is helpful for putting code in place that should only be executed in the absence of exceptions.

python
try:
result = 10
except ZeroDivisionError:
print(“Error: Division by zero is not allowed.”)
else:
print(f”The result is: {result}”)
- try block: The code inside the ‘try’ block is the main code that may raise an exception. In this case, it attempts to perform the division ‘10 / 2’, which is a straightforward calculation.
- ‘except ZeroDivisionError:’ block: This block is executed if a ‘ZeroDivisionError’ occurs in the corresponding try block. In this case, it prints an error message indicating that division by zero is not allowed.
- ‘else’ block: This block is executed if no exception occurs in the ‘try’ block. In this case, it prints the result of the division.
Here’s how the output would look for this specific code:

python
The result is: 5.0
Because the division 10 / 2 is valid and does not result in a division by zero.
Handling Multiple Exceptions
Handling Multiple Exceptions in a Single Except Block
One of Python’s most useful features is the ability to Handle multiple exceptions in a single except block. This lets you write code more efficiently and respond differently to various kinds of problems. You can handle multiple exceptions in a single except block by specifying them as a tuple.

python
try:
value = int(“abc”) # This will raise a ValueError
except (ValueError, TypeError):
print(“Error: Unable to convert the string to an integer.”)
- If the conversion in the try block (int(“abc”)) raises a ValueError or TypeError, the except block is executed.
- The except block prints an error message indicating that the string cannot be converted to an integer.
When you want to deliver personalized error messages or handle failures in a specific manner, this kind of error handling comes in handy.
Raising Exceptions
The Raise Statement and Its Usage
It is possible to manually raise exceptions using the raise statement. When you wish to indicate a mistake under certain circumstances, this might be helpful.

python
if x < 0:
raise ValueError(“x should be a non-negative number”)
In this code:
- ‘if x < 0:’: This is a conditional statement that checks whether the value of variable x is less than 0.
- ‘raise ValueError(“x should be a non-negative number”)’: If the condition is true (i.e., if x is less than 0), a ValueError exception is explicitly raised with a custom error message.
Creating and Raising Custom Exceptions
You can create custom exception classes by inheriting them from the built-in Exception class. This allows you to define your own application-specific exceptions.

python
class CustomError(Exception):
def __init__(self, message=”This is a custom exception”):
self.message = message
super().__init__(self.message)
def example_function(value):
if value < 0:
raise CustomError(“Negative values are not allowed”)
try:
user_input = int(input(“Enter a number: “))
example_function(user_input)
except CustomError as ce:
print(f”CustomError caught: {ce}”)
except ValueError as ve:
print(f”ValueError caught: {ve}”)
except Exception as e:
print(f”An unexpected error occurred: {e}”)
In this example:
- We define a custom exception called CustomError by inheriting from the built-in Exception class.
- The __init__ method is used to customize the exception with a message. The super().__init__(self.message) line ensures that the base class (Exception) is properly initialized.
- The example_function function checks if the provided value is negative. If it is, it raises the CustomError exception with a specific message.
- In the try block, we attempt to get user input, convert it to an integer, and call example_function. If a CustomError or ValueError occurs, it will be caught in the respective except blocks and handled accordingly.
- The except Exception as e block catches any other unexpected exceptions.
Cleanup Actions with Finally
In Python, the finally block is used to define cleanup actions that must be executed regardless of whether an exception occurs or not. This ensures that certain actions, typically related to cleanup, such as closing files or releasing resources will always be performed. The finally block is commonly used in conjunction with the try and except blocks.

python
def example_function(value):
try:
result = 10 / value
print(f”Result: {result}”)
except ZeroDivisionError:
print(“Cannot divide by zero!”)
finally:
print(“This will always be executed, regardless of exceptions.”)
# Example usage
try:
user_input = float(input(“Enter a number: “))
example_function(user_input)
except ValueError:
print(“Invalid input. Please enter a valid number.”)
In this example:
- The example_function function attempts to perform a division operation within the try block.
- If a ZeroDivisionError occurs (e.g., if the user enters 0), the corresponding except block is executed, and a message is printed.
- The finally block contains code that will always be executed, regardless of whether an exception occurred or not. In this case, it prints a message indicating that it will always be executed.
When you run this script, regardless of the input you provide, the finally block will be executed, demonstrating its use for cleanup actions.
Conclusion
In summary, proficiency in Python’s exception handling is essential for developing code that is both resilient and dependable. The try-except block, complemented by else and finally, furnishes a potent framework for gracefully managing errors and executing necessary cleanup procedures. Whether addressing predefined or customized exceptions, integrating exception handling into your code not only fortifies its reliability but also elevates the user experience to a higher standard.