A Guide to Python Operators, Loops and Conditionals
Programming serves as the backbone of the digital world, enabling us to create sophisticated software and applications. At the core of every programming language lie fundamental concepts such as operators, expressions, loops, and conditional statements. In this blog post, we’ll embark on a journey to demystify these concepts, shedding light on their significance in the realm of programming.
A Deep Dive into Operators and Expressions in Programming
Operators:
Operators are the building blocks of expressions, allowing us to perform operations on variables and values. They can be categorized into various types:
- Arithmetic Operators: Arithmetic operators are used to perform mathematical operations on numeric values. Here are the basic arithmetic operators in Python
- Addition (+) – Adds two numbers
- Subtraction (-) – Subtracts the right operand from the left operand
- Multiplication (*) – Multiplies two numbers
- Division (/) – Divides the left operand by the right operand
- Modulus (%) – Returns the remainder of the division of the left operand by the right operand
Example:

python
# Arithmetic operators are used to perform mathematical operations on numeric values. Here are the basic arithmetic operators in Python.
result = 10 + 5 * 2
# Output: 20
2. Comparison Operators: Comparison operators in Python are used to compare values. They return a Boolean result, either True or False, based on whether the comparison is true or false. Here are the common comparison operators in Python:
- == (Equal to) – Checks if two values are equal
- != (Not equal to) – Checks if two values are not equal
- < (Less than) – Checks if the left operand is less than the right operand
- > (Greater than) – Checks if the left operand is greater than the right operand
- <= (Less than or equal to) – Checks if the left operand is less than or equal to the right operand
- >= (Greater than or equal to) – Checks if the left operand is greater than or equal to the right operand
Example:

python
# Comparison operators in Python are used to compare values. They return a Boolean result, either True or False, based on whether the comparison is true or false.
x = 5
y = 10
is_equal = x == y
# Output: False
3. Logical Operators: Logical operators in Python are used to combine and manipulate Boolean values. These operators allow you to perform logical operations on one or more Boolean values. Here are the main logical operators in Python
- and (Logical AND) – Returns True if both operands are True, otherwise returns False
- or (Logical OR) – Returns True if at least one of the operands is True, otherwise returns False
- not (Logical NOT) – Returns True if the operand is False, and False if the operand is True.
Example:

python
# Logical operators in Python are used to combine and manipulate Boolean values. These operators allow you to perform logical operations on one or more Boolean values.
a = True
b = False
result = a and b
# Output: False
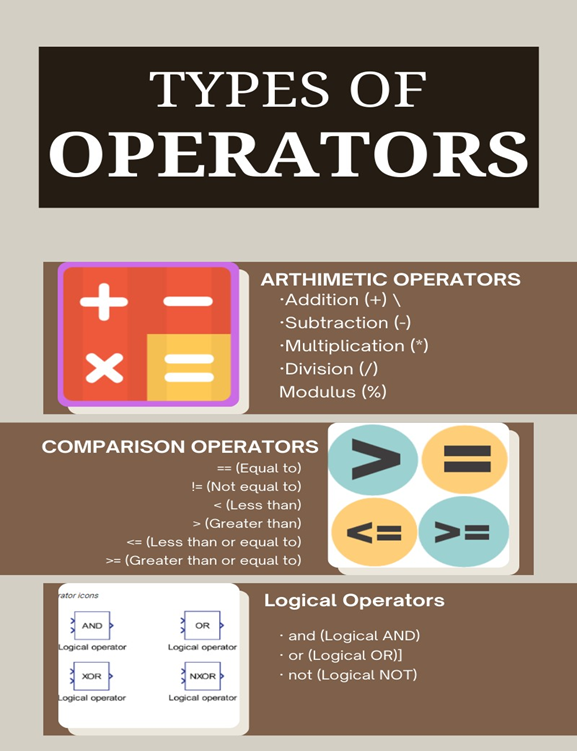
Expressions:
Expressions are combinations of variables, values, and operators that, when evaluated, result in a value. They are the backbone of any programming language, allowing us to perform calculations and make decisions based on conditions.
Example:

python
x = 10
y = 5
result = x + y * 2
print (result)
# Output: 20
Understanding Conditional Statements & Loops:
Understanding control flow is fundamental to programming, and in Python, two key constructs play a pivotal role in controlling the flow of your program: conditional statements and loops. we’ll explore these constructs and provide practical examples to help you master control flow in Python.
Conditional Statements (if, elif, else):
Conditional statements allow you to make decisions in your code based on certain conditions. They follow a straightforward syntax:
- if condition: Code to execute if the condition is True
- elif another_condition: Code to execute if the previous condition is False and this one is True
- else: Code to execute if none of the previous conditions are True
Example:

python
Marks = float(input(‘Enter Your Marks’))
# if condition: Code to execute if the condition is True
if Marks < 20:
print(“You are fail.”)
# elif another_condition: Code to execute if the previous condition is False and this one is True
elif 20 <= Marks < 80:
print(“You are passed with B Grade”)
# else: Code to execute if none of the previous conditions are True
else :
print(“You are passed with A Grade”)
Here, the program checks the Marks variable and prints a message based on the Marks range.
Looping Through Iterables:
Loops are used for iterating through sequences like lists, strings, or ranges. Python supports two main types of loops: for and while.
1. For Loop:
The ‘for’ loop iterates over a sequence (such as a list) and executes a block of code for each element.

python
Thinknyx = [‘Devops’,‘ Cloud Computing’,‘AI automation’]
for courses in Thinknyx:
print(f’We provide {courses} courses’ )
This loop prints a message for each course in the list provided by Thinknyx.
2. While Loop:
The while loop continues executing a block of code as long as a specified condition is true.

python
Thinknyx = [‘Devops’,‘Cloud Computing’,‘AI automation’]
course = 0
while course < len(Thinknyx):
print(f’We provide {Thinknyx[course]} courses’ )
course += 1
This loop prints the Thinknyx variable until it reaches the length of the list.
Loop Control Statements:
Python also provides loop control statements that you can use within loops:
- break: Terminates the loop prematurely.
- continue: Skips the rest of the code inside the loop for the current iteration and moves to the next iteration.
The Conclusion
In conclusion, operators, expressions, loops, and conditional statements form a cohesive ensemble, each playing a unique role in the symphony of programming. Mastering these fundamentals is not just about understanding syntax; it’s about composing elegant and efficient solutions to real-world problems. Like any art form, coding requires practice, experimentation, and a deep appreciation for the nuances of the craft.
As you embark on your coding journey, remember that these fundamental concepts are your companions. They are the notes, rhythms, and harmonies that you’ll arrange to create your masterpiece. With each line of code, you refine your skills, gaining the ability to craft solutions that are not only functional but also elegant and maintainable.
So, keep coding, keep exploring, and let the symphony of your creations resonate in the digital landscape.
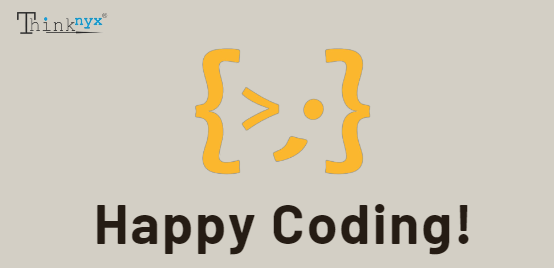