Python’s adaptability extends to many disciplines, including file manipulation. Handling files is an essential skill for any developer since it is the foundation of data storage, retrieval, and processing. In this blog, we will look at the foundations of interacting with files in Python, looking at the methods and functions that allow developers to interface with data files in a natural way.
Why do you need File Handling?
File Handling is required for a number of reasons, the most essential of which are data storage, retrieval, and manipulation. Here are a few of the primary reasons why file management is so crucial in programming:
- Data Persistence: File Handling allows you to save data indefinitely. Data saved in variables is destroyed when your program stops, but by saving it to a file, you may save it for future use.
- Data Sharing: Files are used as a standard medium for data transmission across programs. This procedure allows for smooth communication whether it’s receiving input from a file or sending output to a file.
- Configuration Management: Many applications save configuration parameters in files. File handling allows for the recovery and updating of these settings, offering a flexible means to modify a program’s behaviour.
- Data Analysis: When working with massive datasets, file management is critical. The file-handling features of Python make it easier to read, process, and analyse large amounts of data.
Below image is incomplete without examples of each
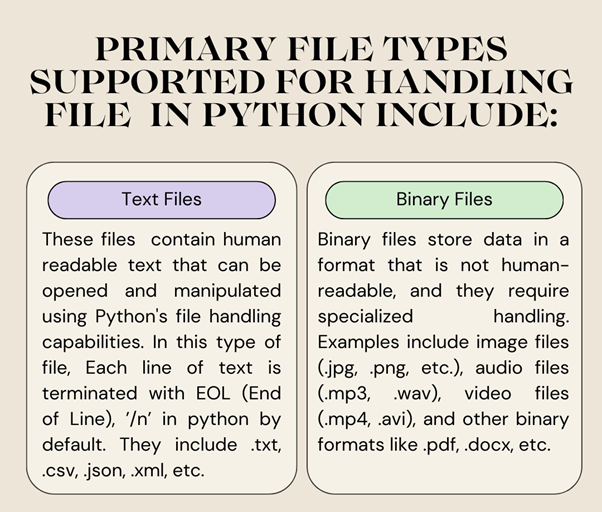
File Operations Using Python — CRUD Operation in Python
In Python, file handling consists of a series of core actions known as CRUD, which stands for Create, Read, Update, and Delete. These operations serve as the foundation for dealing with files, allowing you to interact with data in a variety of ways.
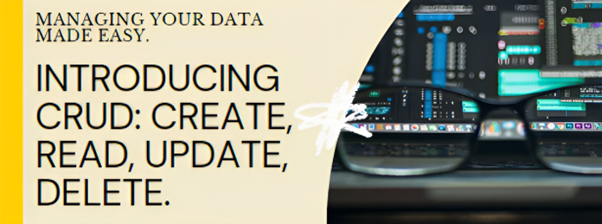
Create (C): Creating a File
The open() method is used to create a file in Python. The open() method allows you to open a file in a variety of modes, including ‘w’ (write), which is typically used to create a new file. Here is a simple example:

python
# Open a file in write mode (‘w’)
with open(‘new_file.txt’, ‘w’) as file:
# Write content to the file
file.write(‘Hello, this is a new file created in Python!’)
Let’s break down the code:
- open(‘new_file.txt’, ‘w’): This section of code starts the file ‘new_file.txt’ in write mode (‘w’). Python will create the file if it does not already exist. If the file already exists, opening it in write mode will truncate its content, resulting in a file that is effectively empty.
- with open(…) as file: The with statement ensures that the file is correctly closed when the code block is run. To minimise potential problems, this is a best practise in Python for file management.
- file.write(‘Hello, this is a new file created in Python!’): The write() function is used within the with block to add specified text to the file. The string ‘Hello, this is a new file created in Python!’ is written to the file in this example.
Keep in mind that file handling procedures may throw exceptions, therefore it’s best to use the with a statement to handle these actions gracefully by ensuring that the file is correctly closed, even if an exception happens during the process.
In Python, the ‘x’ mode is a file access mode used in file handling operations when opening a file for writing but specifically for creating a new file. This mode is exclusive to Python 3.3 and later versions.
When using the ‘x’ mode to open a file:
- If the file does not exist, it creates a new file for writing.
- If the file already exists, it raises a ‘FileExistsError’.
Here’s an example of using the ‘x’ mode in file handling:

python
with open(‘new_file1.txt’, ‘x’) as file:
file.write(“This is a new file created using ‘x’ mode in Python file handling.”)
print(“New file created”)
In this example:
- open(‘new_file.txt’, ‘x’) tries to open/create a file named new_file.txt in ‘x’ mode for writing.
- If new_file.txt already exists, it will raise a FileExistsError.
- If new_file.txt does not exist, it will create a new file and allow writing to it.
NOTE: Remember, the ‘x’ mode is particularly useful when you want to ensure that you’re creating a new file and avoid accidentally overwriting existing files.
Read (r): Creating a File
Reading a file in Python is a typical task that may be accomplished by using the open() method in the ‘r’ (read) mode. Here’s a simple example:

python
# Open a file in read mode (‘r’)
with open(‘example.txt’, ‘r’) as file:
# Read content from the file
content = file.read()
print(content)
Let’s break down the code:
- open(‘example.txt’, ‘r’): This section of code starts the file ‘example.txt’ in read mode (‘r’). If the file does not exist, a FileNotFoundError will be thrown.
- with open(…) as file:: The with statement ensures that the file is correctly closed when the code block is run. To avoid potential problems, this is excellent file management practise.
- content = file.read(): The read() function is used within the with block to read the whole contents of the file and save it in the variable ‘content’. You may also read one line at a time by using methods like readline(). print(content): Finally, the file’s contents is printed to the console.
Update (U): Modifying a File
In Python, updating a file entails editing its current content. This is commonly done with the open() function in the ‘a’ (append) or ‘w’ (write) modes.
Let’s investigate both approaches:
Append Mode (‘a’): It opens a file for appending text at the end of the file. It doesn’t erase existing content.

python
# Open a file in append mode (‘a’)
with open(‘existing_file.txt’, ‘a’) as file:
# Append new content to the file
file.write(‘\nThis content is appended!’)
The file ‘existing_file.txt’ is opened in append mode in this example. The ‘\n’ is an escape sequence which denotes a new line, therefore the added information begins on a new line.
Overwrite Mode (‘w’):

python
# Open a file in write mode (‘w’)
with open(‘existing_file.txt’, ‘w’) as file:
# Overwrite the existing content with new content
file.write(‘This content will overwrite the existing content.’)
In this case, the file is opened in write mode (‘w’), and the previous content of ‘existing_file.txt’ is completely overwritten with the new text supplied.
Delete (D): Removing a File
In Python, deleting a file means removing it from the file system. For file deletion, the os.remove() method is widely used. To avoid mistakes, it’s a good idea to check whether a file exists before deleting it.

python
import os
file_to_delete = ‘file_to_be_deleted.txt’
# Check if the file exists
if os.path.exists(file_to_delete):
# Remove the file
os.remove(file_to_delete)
print(f'{file_to_delete} has been deleted.’)
else:
print(f'{file_to_delete} does not exist.’)
In this case:
- path.exists(file_to_delete): This section determines whether or not the file supplied by the variable file_to_delete exists in the current directory.
- remove(file_to_delete): The os.remove() method is used to delete the file if it exists.
- print(f’file_to_delete was successfully deleted.’): To confirm that the file has been destroyed, a message is printed.
If the file does not exist, a message is printed indicating that it cannot be located.
Replace ‘file_to_be_deleted.txt’ with the name of the file you wish to remove. Also, use caution while performing file deletion procedures, since they are irrevocable, and destroyed files cannot be retrieved easily. Before conducting any deletion activities, double-check the file name and path.
Best Practices in File Handling
When interacting with files in Python, following best practices promotes efficient and error-free programming. Here are several standard practices for file management in Python:
- Use ‘with open()’ Statement: When opening files, always use the with statement. It provides good resource management and automatically closes the file when finished.
- Check File Existence: To avoid issues, check if the file exists before performing activities such as deletion.
- Exception Handling: File operations may result in exceptions. Implement suitable error handling to manage errors that may arise during file handling gently.
Following these best practices will guarantee that your file handling code is robust, legible, and error-free. Proper file management is required for developing dependable and maintainable Python programs.
In Conclusion
File handling is an essential part of programming that allows developers to interface with external files for activities such as data storage, retrieval, and modification. Python provides a robust and powerful collection of file management utilities, allowing developers to easily create, read, update, and remove files.
Developers can create robust and error-resistant file-handling code by following best practices such as using the with statement for proper resource management, checking for file existence before operations, handling exceptions gracefully, specifying file encoding, using absolute paths, and being cautious with write modes.
Learning how to handle files in Python is essential for a variety of applications, ranging from data persistence and configuration management to data sharing and analysis. Understanding and adopting these file handling techniques can help you write cleaner, more maintainable, and dependable code, whether you are a newbie or an experienced developer.