Introduction To Modules
Python, a versatile and powerful programming language, offers a feature known as modules, which allows developers to organize their code efficiently. A module is a file containing Python definitions and statements. It serves as a repository of functions, classes, and variables, providing a structured way to organize code and promote code reuse.
Why Use Modules?
Modules bring several advantages to the key points:
- Organization: Modules facilitate the organization of code by grouping related functionalities.
- Reusability: Code written in a module can be reused in different parts of the program or even in other projects, promoting modularity and reducing redundancy.
- Namespace Management: Modules provide a namespace, preventing naming conflicts and allowing developers to maintain a clear and coherent naming structure.
- Maintainability: Breaking code into modules makes it easier to understand, maintain, and debug.
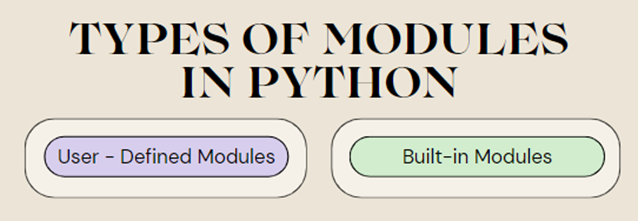
User-defined modules
User-defined modules typically refer to custom modules created by a programmer or user in a Python. The term is commonly used in the context of modular programming, where a program is divided into separate, manageable functions, each responsible for a specific functionality.
Creating Your Modules
Creating a module is as simple as creating a Python file. For instance, if you want to create a module named my_module, you can create a file named my_module.py and define your functions, classes, and variables within it.
Write your Python code:
In your text editor or IDE, create a new file and write the Python code that you want to include in your module. For example:

python
# example_module.py
def greet(name):
return f”Hello, {name}!”
def square(x):
return x ** 2
if __name__ == “__main__”:
print(“This is the main module.”)
In this example,
- In this module, we define two functions: greet and square.
- The if __name__ == “__main__”: block ensures that the code inside it only runs if the module is executed directly and not when it’s imported.
Importing the Entire Module
Modules are Python’s way of organising code, fostering reusability, and maintaining a clean codebase. Let’s take a quick journey into the world of importing modules, a crucial skill for any Pythonista.

python
# main_script.py
# Importing the entire module
import example_module
# Using functions from the module
print(example_module.greet(“India”))
print(example_module.square(5))
Here, we import the entire module using import example_module.
Functions from the module are accessed using the dot notation, such as example_module.greet(“India”).
This illustrates how easy and effective it is to import modules in Python.
Importing Specific Functions or Variables:
The ‘from’ keyword in Python serves as a selective import mechanism, enabling developers to bring specific components from a module into their current namespace. This means you can use those components directly without referencing the module each time.
Let’s explore a simple example of using the ‘from’ keyword to import a specific function from a module.

python
# main_script.py
# Importing specific functions using keywords
from example_module import greet, square
# Using the imported functions directly
print(greet(“Asia”))
print(square(3))
- In this example, we import specific functions (greet and square) from the module using from example_module import greet, square.
- This allows us to use these functions directly in the script without referencing the module name.
In summary, creating a module involves defining functions or classes in a separate file. Importing the entire module is done using import module_name, while importing specific functions is achieved with module_name import function1, function2. This modular approach helps organize code and promotes reusability.
Built-in Module (Standard Python Modules)
Built-in modules in Python are pre-existing modules that come with the Python programming language. These modules provide a wide range of features, making it easier for developers to execute numerous tasks without having to create everything from scratch. These modules are part of the Python Standard Library, a collection of modules and packages extending the language’s capabilities.
If you want to list all the methods of a built-in module in Python. Using the dir() function, you can pass ‘module_name’ as parameter , and get the list of all the methods and variables. Here’s an example using the math module:

python
import math
print(dir(math))
This command will output a list of all the characteristics (including methods) of the provided module. Keep in mind that this includes not only methods, but also variables and classes specified in the module.
Replace ‘math’ with the module name you wish to explore.
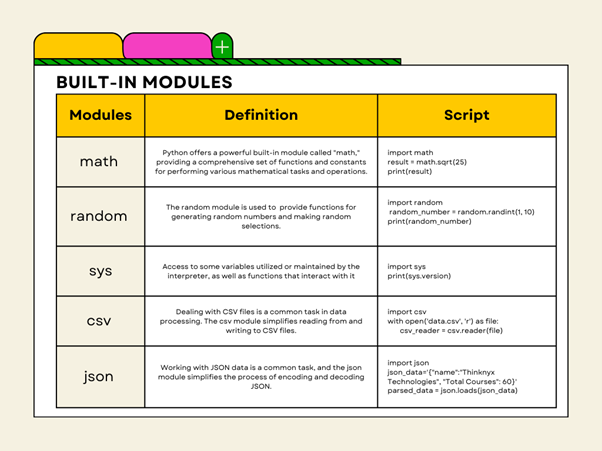
Renaming a module (Using 'as' keyword)
In Python, when you import a module, you may rename it using the ‘as’ keyword. This can be beneficial for giving module a shorter or more convenient name. The following is the syntax for renaming a module:

python
import module_name as new_name
Here’s an example using the ‘math’ module:

python
import math as m
result = m.sqrt(25)
print(result) # Output: 5.0
In this example, ‘math’ is imported with the alias ‘m’. From this point forward in the code, you can use ‘m’ instead of ‘math’ to reference functions and variables from the module.
Conclusion
To sum up, modules are essential to Python programming because they offer a strong framework for code reuse, organisation, and maintainability. Through the division of functionality into modular parts, developers may create codebases that are legible, efficient, and scalable.
Python’s robust third-party package ecosystem and large standard library allow developers to tackle a wide range of jobs without having to start from scratch. This promotes a collaborative atmosphere where code reuse and sharing are encouraged in addition to speeding up development.